-
Notifications
You must be signed in to change notification settings - Fork 87
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
Merge pull request #1534 from vtexdocs/EDU-13483-faststore-analytics
EDU-13483: FastStore Analytics
- Loading branch information
Showing
3 changed files
with
171 additions
and
0 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,58 @@ | ||
--- | ||
title: "Analytics" | ||
--- | ||
|
||
The Analytics module manages events on a website. The module is composed of two main functions: | ||
|
||
- [`sendAnalyticsEvent`](https://developers.vtex.com/docs/guides/faststore/analytics-send-analytics-event): Fires events in the browser. Events fired using this function are shared only with the website's origin. The event is wrapped and sent to the [Window object](https://developer.mozilla.org/en-US/docs/Web/API/Window) via standard `postMessage` calls. | ||
- [`useAnalyticsEvent`](https://developers.vtex.com/docs/guides/faststore/analytics-use-analytics-event): Intercepts fired events and usually communicates with an analytics provider. | ||
|
||
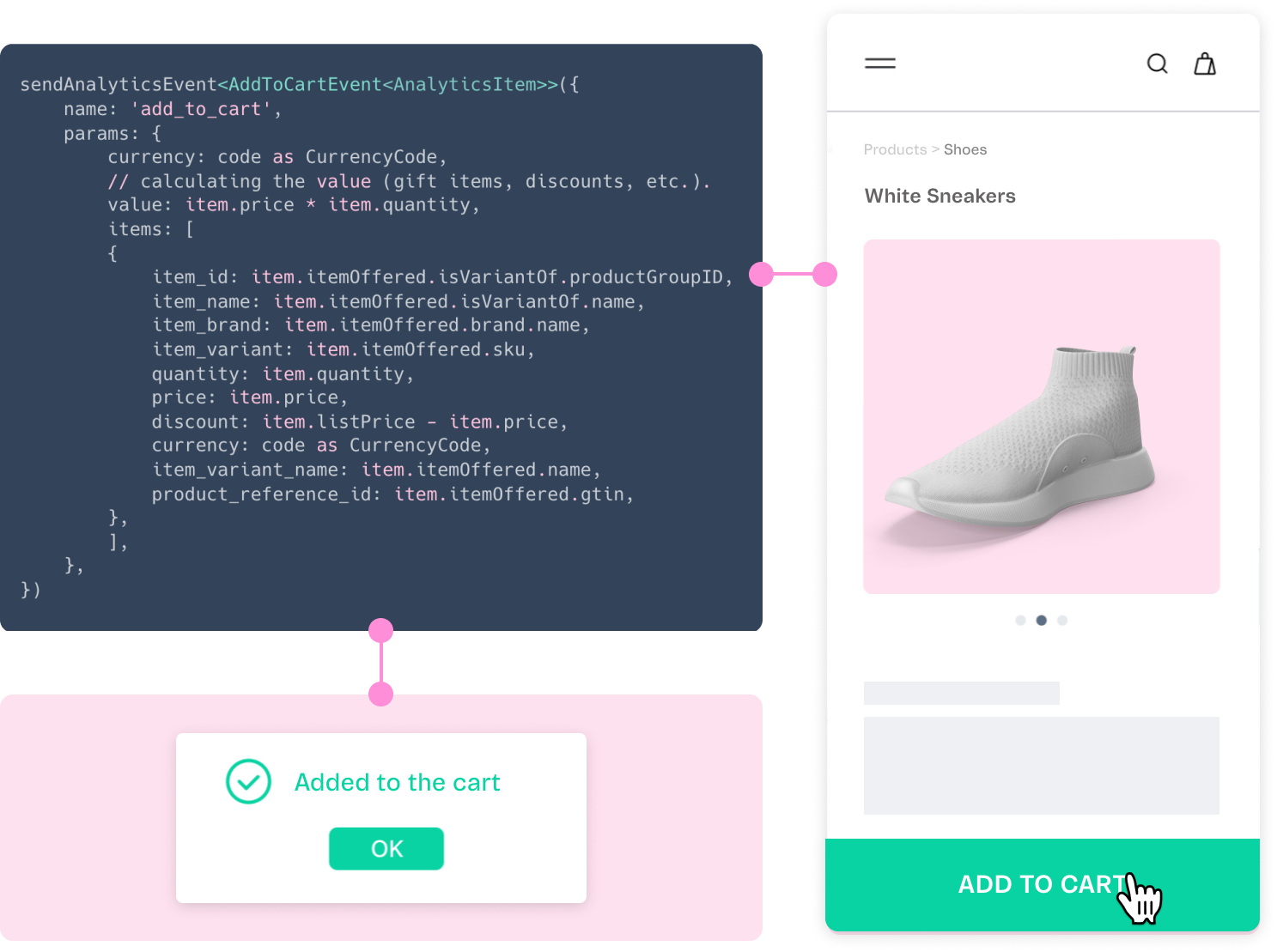 | ||
|
||
> ⚠️ Events sent via the Analytics module are not automatically sent to any analytics provider (e.g., Google Analytics). To connect with a provider, configure the [`useAnalyticsEvent`](https://developers.vtex.com/docs/guides/faststore/analytics-use-analytics-event) hook. | ||
The Analytics module supports sending and receiving different events, allowing you to implement custom event types and override default ones. | ||
|
||
Additionally, the Analytics module offers built-in [ecommerce event](https://support.google.com/analytics/answer/14434488?hl=en) types based on the [Google Analytics 4 (GA4) data model](https://developers.google.com/analytics/devguides/collection/ga4/reference/events). | ||
|
||
## List of native event types | ||
|
||
The Analytics module comes with native types based on [ecommerce events](https://support.google.com/analytics/answer/14434488?hl=en). All event types are available for use and extension. Here is a list of events natively supported by the Analytics module: | ||
|
||
| Type | Parameters | | ||
|--------|-----------------| | ||
|`add_payment_info`|`currency`, `value`, `coupon`, `payment_type`, and `items`| | ||
|`add_shipping_info`|`currency`, `value`, `coupon`, `shipping_tier`, and `items`| | ||
|`add_to_cart`|`currency`, `value`, and `items`| | ||
|`add_to_wishlist`|`currency`, `value`, and `items`| | ||
|`begin_checkout`|`currency`, `value`, `coupon`, and `items`| | ||
|`login`|`method`| | ||
|`page_view`|`page_title`, `page_location`, and `send_page_view`| | ||
|`purchase`|`currency`, `transaction_id`, `value`, `affiliation`, `coupon`, `shipping`, `tax`, and `items`| | ||
|`refund`|`currency`, `transaction_id`, `value`, `affiliation`, `coupon`, `shipping`, `tax`, and `items`| | ||
|`remove_from_cart`|`currency`, `value`, and `items`| | ||
|`search`|`search_term`| | ||
|`select_item`|`item_list_id`, `item_list_name`, and `items`| | ||
|`select_promotion`|`item_list_id`, `item_list_name`, and `items`| | ||
|`share`|`method`, `content_type`, and `item_id`| | ||
|`signup`|`method`, `content_type`, and `item_id`| | ||
|`view_cart`|`currency`, `value`, and `items`| | ||
|`view_item`|`currency`, `value`, and `items`| | ||
|`view_item_list`|`item_list_id`, `item_list_name`, and `items`| | ||
|`view_promotion`|`items`| | ||
|
||
Each event exports at least two types: one for the event parameters and one for the event type itself. For example, the `add_to_cart` event has two exported types: `AddToCartParams<T extends Item = Item>` and `AddToCartEvent<T extends Item = Item>`. | ||
|
||
Some types are [common](https://github.com/vtex/faststore/blob/main/packages/sdk/src/analytics/events/common.ts) to all events, such as the `Item` type. These types are particularly useful when overriding `Item` properties or a whole `Item` itself. | ||
|
||
## Google Analytics 4 | ||
|
||
Google Analytics is the industry-leading analytics solution that most ecommerce websites use, and the Analytics module was designed with this in mind. All the helper functions and hooks in the module use the [Google Analytics 4 (GA4) data model](https://developers.google.com/analytics/devguides/collection/ga4/reference/events) by default. This allows you to use [code hinting](#code-hinting) tools to receive suggestions of GA4 events and their recommended properties while coding. | ||
|
||
To send the events to Google Analytics, use the [`useAnalyticsEvent`](https://developers.vtex.com/docs/guides/faststore/analytics-use-analytics-event) hook. The hook must listen to events from [`sendAnalyticsEvents`](https://developers.vtex.com/docs/guides/faststore/analytics-send-analytics-event) and add them to the `dataLayer` (recommended for Google Tag Manager) or call the `gtag` function directly (for `gtag` script implementations). | ||
|
||
## Code hinting | ||
|
||
By leveraging the type definitions in the Analytics module, you can use [IntelliSense](https://code.visualstudio.com/docs/editor/intellisense) suggestions for code hinting. | ||
|
||
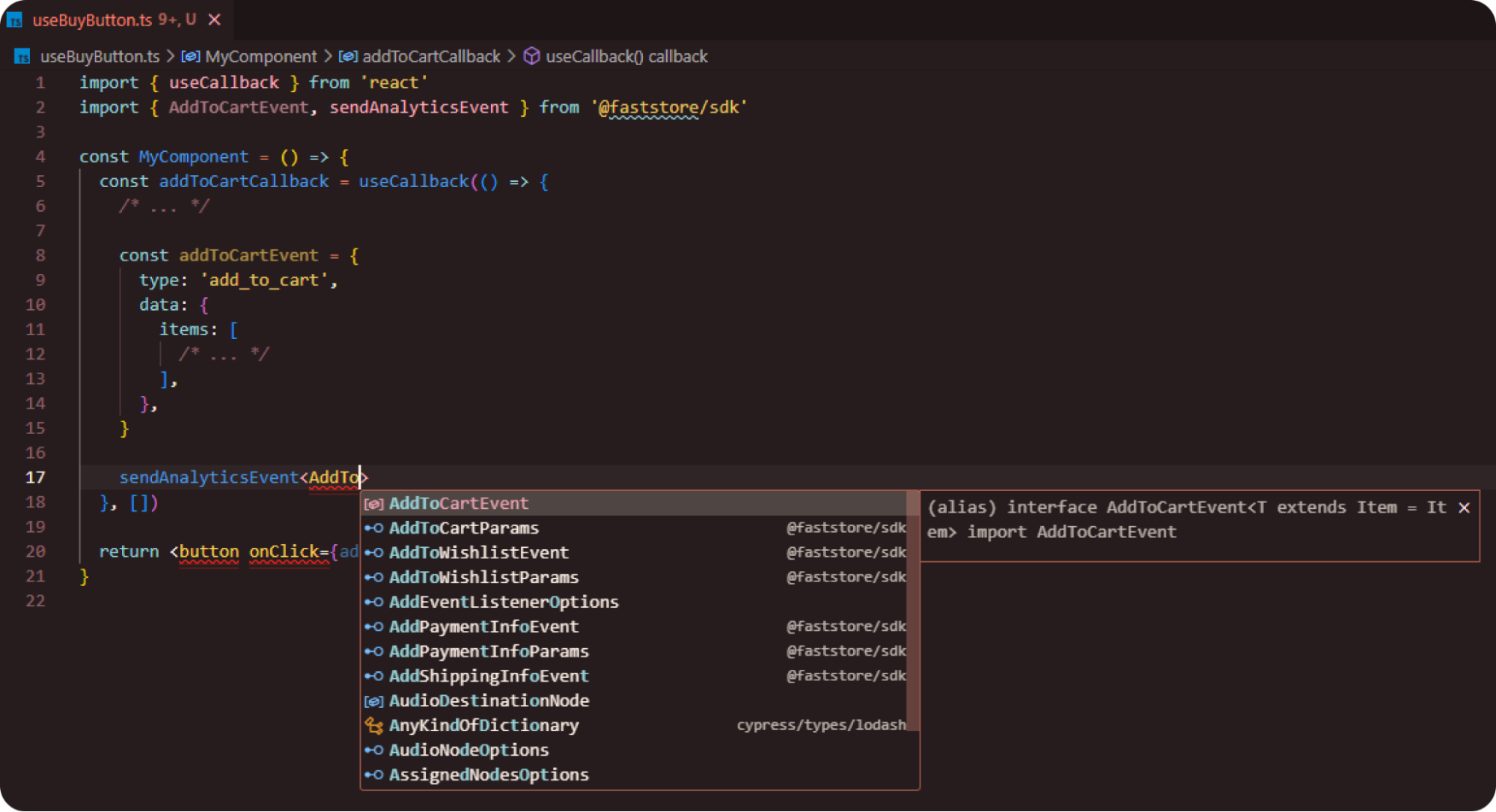 |
71 changes: 71 additions & 0 deletions
71
docs/faststore/docs/sdk/analytics/send-analytics-event.mdx
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,71 @@ | ||
--- | ||
title: "sendAnalyticsEvent" | ||
--- | ||
|
||
The `sendAnalyticsEvent` function triggers events in the browser, following the [Google Analytics 4 (GA4) data model](https://developers.google.com/analytics/devguides/collection/ga4/reference/events). This function is primarily used for ecommerce tracking but also supports custom events, serving as a centralized point for managing events. | ||
|
||
{ /* Remember to add a link to 'custom events' once we have the following task done: EDU-13545 */ } | ||
|
||
> ⚠️ `sendAnalyticsEvent` doesn't send events to any analytics provider. To intercept events triggered by this function, use the [`useAnalyticsEvent`](https://developers.vtex.com/docs/guides/faststore/analytics-use-analytics-event) hook. | ||
## Import | ||
|
||
```tsx | ||
import { sendAnalyticsEvent } from '@faststore/sdk' | ||
``` | ||
|
||
## Usage | ||
|
||
In the component related to the event, declare a callback function. In this function, define an event object with the desired event type (example: `add_to_cart`) and call `sendAnalyticsEvent`. Then, pass the related event as an argument. Finally, call the callback function in the event trigger (example: `onClick`). | ||
|
||
Consider the following example of an `add_to_cart` event triggered by the `button` component: | ||
|
||
```tsx | ||
import { useCallback } from 'react' | ||
import { sendAnalyticsEvent } from '@faststore/sdk' | ||
|
||
const MyComponent = () => { | ||
const addToCartCallback = useCallback(() => { | ||
import('@faststore/sdk').then(({ sendAnalyticsEvent }) => { | ||
/* ... */ | ||
|
||
const addToCartEvent = { | ||
name: 'add_to_cart', | ||
params: { | ||
items: [ | ||
/* ... */ | ||
], | ||
}, | ||
} | ||
|
||
sendAnalyticsEvent(addToCartEvent) | ||
}) | ||
}, []) | ||
|
||
return <button onClick={addToCartCallback}>Add to cart</button> | ||
} | ||
``` | ||
|
||
Check the list of [available types](https://developers.vtex.com/docs/guides/faststore/analytics-overview#list-of-native-event-types). | ||
|
||
## Exporting custom event types with generics | ||
|
||
The `sendAnalyticsEvent` function supports generics, enabling you to extend default types or modify existing ones. This approach provides type-checking and code suggestions for your custom event properties. | ||
|
||
Consider the following example of providing a custom type reference for `sendAnalyticsEvent`: | ||
|
||
```tsx | ||
import { sendAnalyticsEvent } from '@faststore/sdk' | ||
|
||
interface CustomEvent { | ||
name: 'custom_event' | ||
params: { | ||
customProperty?: string | ||
} | ||
} | ||
|
||
/** | ||
* By passing CustomEvent as a generic type to sendAnalyticsEvent, you'll receive code hints for all properties of this type, including params subfields. | ||
*/ | ||
sendAnalyticsEvent<CustomEvent>(/* ... */) | ||
``` |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,42 @@ | ||
--- | ||
title: "useAnalyticsEvent" | ||
--- | ||
|
||
The `useAnalyticsEvent` hook intercepts both native and custom events triggered by the [`sendAnalyticsEvent`](https://developers.vtex.com/docs/guides/faststore/analytics-send-analytics-event) function. The hook automatically detects the events sent by `sendAnalyticsEvent` and provides a response to them. | ||
|
||
{ /* Remember to add a link to 'custom events' once we have the following task done: EDU-13545 */ } | ||
|
||
## Import | ||
|
||
```tsx | ||
import type { AnalyticsEvent } from '@faststore/sdk' | ||
import { useAnalyticsEvent } from '@faststore/sdk' | ||
``` | ||
|
||
## Usage | ||
|
||
The `useAnalyticsEvent` hook accepts a callback function that runs whenever the `sendAnalyticsEvent` is triggered. The callback function receives the event that triggered its execution as an argument. | ||
|
||
Ideally, you should use the `useAnalyticsEvent` hook to transmit events to the analytics provider of your choice (e.g., Google Analytics). | ||
|
||
```tsx | ||
import type { AnalyticsEvent } from '@faststore/sdk' | ||
import { useAnalyticsEvent } from '@faststore/sdk' | ||
|
||
export const AnalyticsHandler = () => { | ||
useAnalyticsEvent((event: AnalyticsEvent) => { | ||
/** | ||
* This is where you can send events to your analytics provider | ||
* | ||
* Example: | ||
* window.dataLayer.push({ event: event.name, ecommerce: event.params }) | ||
*/ | ||
}) | ||
|
||
/* ... */ | ||
} | ||
``` | ||
|
||
## Events from external libraries | ||
|
||
External libraries can also send events via the Analytics module, which means you might come across unexpected events being intercepted by `useAnalyticsEvent`. This is usually not an issue, as most common analytics providers don't break if you send them meaningless data. However, if that's the case, filter events by name to ensure only the desired events are sent to the provider. |