-
Notifications
You must be signed in to change notification settings - Fork 24.4k
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
Fix medium font weights for TextInput on Android (#26434)
Summary: When using a medium (500) font weight on Android the wrong weight is used for the placeholder and for the first few seconds of input (before it gets text back from JS). To fix it I refactored the way we handle text styles (family, weight, style) to create a typeface to be more like the `Text` component. Since all these 3 props are linked and used to create the typeface object it makes more sense to do it at the end of setting props instead of in each prop handler and trying to recreate the object without losing styles set by other prop handlers. Do do that we now store fontFamily, fontStyle and fontWeight as ivar of the ReactEditText class. At the end of updating prop if any of those changed we recreate the typeface object. This doesn't actually fix the bug but was a first step towards it. There were a bunch of TODOs in the code to remove duplication between `Text` and `TextInput` for parsing and creating the typeface object. To do that I simply moved the code to util functions in a static class. Once the duplication was removed the bug was fixed! I assume proper support for medium font weights was added for `Text` but not in the duplicated code for `TextInput`. ## Changelog [Android] [Fixed] - Fix medium font weights for TextInput on Android Pull Request resolved: #26434 Test Plan: Tested in my app and in RNTester that custom styles for both text and textinput all seem to work. Repro in RNTester: ```js function Bug() { const [value, setValue] = React.useState(''); return ( <TextInput style={[ styles.singleLine, {fontFamily: 'sans-serif', fontWeight: '500', fontSize: 32}, ]} placeholder="Sans-Serif 500" value={value} onChangeText={setValue} /> ); } ``` Before: 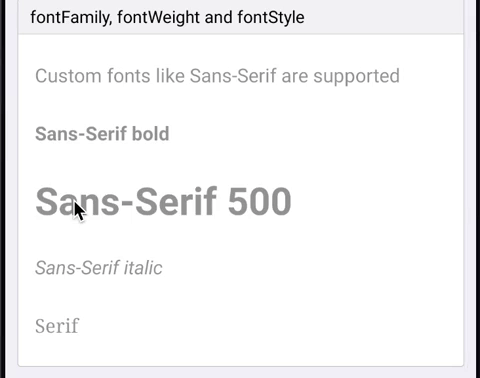 After: 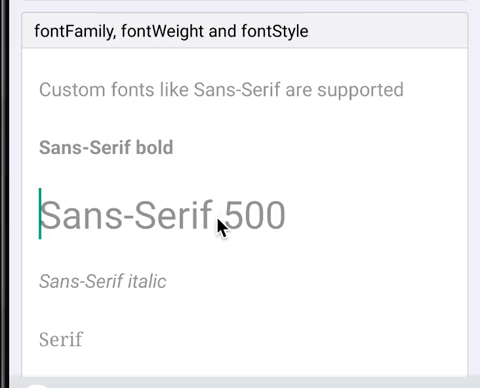 Reviewed By: mmmulani Differential Revision: D17468825 Pulled By: JoshuaGross fbshipit-source-id: bc2219facb94668551a06a68b0ee4690e5474d40
- Loading branch information
1 parent
0cafa0f
commit 8b9f790
Showing
7 changed files
with
181 additions
and
134 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
87 changes: 87 additions & 0 deletions
87
ReactAndroid/src/main/java/com/facebook/react/views/text/ReactTypefaceUtils.java
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,87 @@ | ||
/** | ||
* Copyright (c) Facebook, Inc. and its affiliates. | ||
* | ||
* <p>This source code is licensed under the MIT license found in the LICENSE file in the root | ||
* directory of this source tree. | ||
*/ | ||
package com.facebook.react.views.text; | ||
|
||
import android.content.res.AssetManager; | ||
import android.graphics.Paint; | ||
import android.graphics.Typeface; | ||
|
||
import androidx.annotation.Nullable; | ||
|
||
public class ReactTypefaceUtils { | ||
public static final int UNSET = -1; | ||
|
||
public static int parseFontWeight(@Nullable String fontWeightString) { | ||
int fontWeightNumeric = | ||
fontWeightString != null ? parseNumericFontWeight(fontWeightString) : UNSET; | ||
int fontWeight = fontWeightNumeric != UNSET ? fontWeightNumeric : Typeface.NORMAL; | ||
|
||
if (fontWeight == 700 || "bold".equals(fontWeightString)) fontWeight = Typeface.BOLD; | ||
else if (fontWeight == 400 || "normal".equals(fontWeightString)) fontWeight = Typeface.NORMAL; | ||
|
||
return fontWeight; | ||
} | ||
|
||
public static int parseFontStyle(@Nullable String fontStyleString) { | ||
int fontStyle = UNSET; | ||
if ("italic".equals(fontStyleString)) { | ||
fontStyle = Typeface.ITALIC; | ||
} else if ("normal".equals(fontStyleString)) { | ||
fontStyle = Typeface.NORMAL; | ||
} | ||
|
||
return fontStyle; | ||
} | ||
|
||
public static Typeface applyStyles(@Nullable Typeface typeface, | ||
int style, int weight, @Nullable String family, AssetManager assetManager) { | ||
int oldStyle; | ||
if (typeface == null) { | ||
oldStyle = 0; | ||
} else { | ||
oldStyle = typeface.getStyle(); | ||
} | ||
|
||
int want = 0; | ||
if ((weight == Typeface.BOLD) | ||
|| ((oldStyle & Typeface.BOLD) != 0 && weight == ReactTextShadowNode.UNSET)) { | ||
want |= Typeface.BOLD; | ||
} | ||
|
||
if ((style == Typeface.ITALIC) | ||
|| ((oldStyle & Typeface.ITALIC) != 0 && style == ReactTextShadowNode.UNSET)) { | ||
want |= Typeface.ITALIC; | ||
} | ||
|
||
if (family != null) { | ||
typeface = ReactFontManager.getInstance().getTypeface(family, want, weight, assetManager); | ||
} else if (typeface != null) { | ||
// TODO(t9055065): Fix custom fonts getting applied to text children with different style | ||
typeface = Typeface.create(typeface, want); | ||
} | ||
|
||
if (typeface != null) { | ||
return typeface; | ||
} else { | ||
return Typeface.defaultFromStyle(want); | ||
} | ||
} | ||
|
||
/** | ||
* Return -1 if the input string is not a valid numeric fontWeight (100, 200, ..., 900), otherwise | ||
* return the weight. | ||
*/ | ||
private static int parseNumericFontWeight(String fontWeightString) { | ||
// This should be much faster than using regex to verify input and Integer.parseInt | ||
return fontWeightString.length() == 3 | ||
&& fontWeightString.endsWith("00") | ||
&& fontWeightString.charAt(0) <= '9' | ||
&& fontWeightString.charAt(0) >= '1' | ||
? 100 * (fontWeightString.charAt(0) - '0') | ||
: UNSET; | ||
} | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Oops, something went wrong.