-
Notifications
You must be signed in to change notification settings - Fork 1
Music Player
Before you begin, make sure that Media Plater service is enabled under the plugin Settings.

You also need to enable Music Player Controller API and fill in the Media Library Usage Descitbiont.
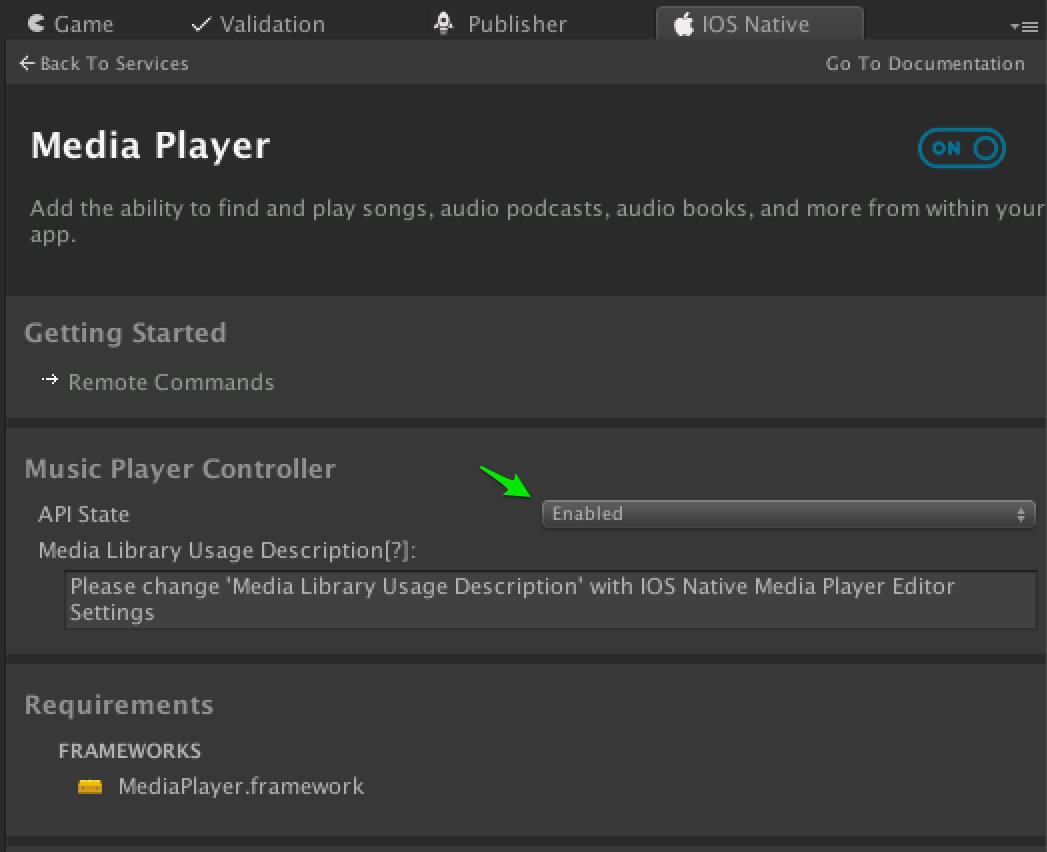
Create an instance of a music player to play media items in your app. There are two types of music player:
-
An application music player plays music locally within your app. It is not aware of the Music app’s now-playing item, nor does it affect the Music app’s state. There are two application music players: ApplicationMusicPlayer and ApplicationQueuePlayer. The application queue player provides greater control over the contents of the queue and is the preferred player.
-
The system music player employs the built-in Music app on your behalf. On instantiation, it takes on the current Music app state, such as the identification of the now-playing item. If a user switches away from your app while music is playing, that music continues to play. The Music app then has your music player’s most recently-set repeat mode, shuffle mode, playback state, and now-playing item.
First of all, you need to create an instance of ISN_MPMusicPlayerController, you can use one of the 3 options, based on your application needs. The ISN_MPMusicPlayerController class is based on iOS MPMusicPlayerController class. Let us know if there is some feature that you are missing comparing to full iOS API
using SA.iOS.MediaPlayer;
...
var player = ISN_MPMusicPlayerController.SystemMusicPlayer;
using SA.iOS.MediaPlayer;
...
var player = ISN_MPMusicPlayerController.ApplicationMusicPlayer;
using SA.iOS.MediaPlayer;
...
var player = ISN_MPMusicPlayerController.ApplicationQueuePlayer;
Once you have an ISN_MPMusicPlayerController instance you can control playback using the class available methods.
The current track info is represented as ISN_MPMediaItem object which is based on MPMediaItem native class. Let us know if there is some feature that you are missing compared to MPMediaItem. The code snippet below shows ho yo retrieve information of the current track from the player.
using SA.iOS.MediaPlayer;
...
var player = ISN_MPMusicPlayerController.SystemMusicPlayer;
ISN_MPMediaItem item = player.NowPlayingItem;
Debug.Log("item.Title: " + item.Title);
Debug.Log("item.Artist: " + item.Artist);
Debug.Log("item.AlbumTitle: " + item.AlbumTitle);
Debug.Log("item.Composer: " + item.Composer);
Debug.Log("item.Genre: " + item.Genre);
Debug.Log("item.Lyrics: " + item.Lyrics);
You can subscribe to the media player playback notification via the Notification Center. You also might want to begin generating playback notifications using the BeginGeneratingPlaybackNotifications method. The code snippet below dementates hot to subscribe to the NowPlayingItemDidChange and PlaybackStateDidChange player events.
using SA.iOS.Foundation;
using SA.iOS.MediaPlayer;
...
var player = ISN_MPMusicPlayerController.SystemMusicPlayer;
player.BeginGeneratingPlaybackNotifications();
var center = ISN_NSNotificationCenter.DefaultCenter;
center.AddObserverForName(ISN_MPMusicPlayerController.NowPlayingItemDidChange,
(ISN_NSNotification notification) => {
Debug.Log("MusicPlayer Now Playing Item Did Change");
});
center.AddObserverForName(ISN_MPMusicPlayerController.PlaybackStateDidChange,
(ISN_NSNotification notification) => {
Debug.Log("MusicPlayer Playback State Did Change");
});
Reliable and high-quality Unity Development service. Let's Talk!
Website | AssetStore | LinkedIn | Youtube | Scripting Reference
- Getting Started
- Authentication
- Game Center UI
- Leaderboards
- Default Leaderboard
- Achievements
- Saving A Game
- Access Point
- iTunes Connect Setup
- StoreKit Initialization
- Purchase flow
- Receipt Validation
- Store Review Controller
- Storefront API
- Subscription Offers