diff --git a/components/doc/breadcrumb/pt/ptdoc.js b/components/doc/breadcrumb/pt/ptdoc.js
new file mode 100644
index 0000000000..197acd4fc1
--- /dev/null
+++ b/components/doc/breadcrumb/pt/ptdoc.js
@@ -0,0 +1,82 @@
+import { BreadCrumb } from '../../../lib/breadcrumb/BreadCrumb';
+import { DocSectionCode } from '../../common/docsectioncode';
+import { DocSectionText } from '../../common/docsectiontext';
+
+export function PTDoc(props) {
+ const items = [{ label: 'Computer' }, { label: 'Notebook' }, { label: 'Accessories' }, { label: 'Backpacks' }, { label: 'Item' }];
+ const home = { icon: 'pi pi-home', url: 'https://primereact.org' };
+
+ const code = {
+ basic: `
+
+ `,
+ javascript: `
+import React from 'react';
+import { BreadCrumb } from 'primereact/breadcrumb';
+
+export default function PTDemo() {
+ const items = [{ label: 'Computer' }, { label: 'Notebook' }, { label: 'Accessories' }, { label: 'Backpacks' }, { label: 'Item' }];
+ const home = { icon: 'pi pi-home', url: 'https://primereact.org' }
+
+ return (
+
+ ({
+ className: props.index === items.length - 1 ? 'font-italic' : undefined
+ })
+ }}
+ model={items}
+ home={home}
+ />
+
+ )
+}
+ `,
+ typescript: `
+import React from 'react';
+import { BreadCrumb } from 'primereact/breadcrumb';
+import { MenuItem } from 'primereact/menuitem';
+
+export default function PTDemo() {
+ const items: MenuItem[] = [{ label: 'Computer' }, { label: 'Notebook' }, { label: 'Accessories' }, { label: 'Backpacks' }, { label: 'Item' }];
+ const home: MenuItem = { icon: 'pi pi-home', url: 'https://primereact.org' }
+
+ return (
+
+ ({
+ className: props.index === items.length - 1 ? 'font-italic' : undefined
+ })
+ }}
+ model={items}
+ home={home}
+ />
+
+ )
+}
+ `
+ };
+
+ return (
+ <>
+
+
+ ({
+ className: props.index === items.length - 1 ? 'font-italic' : undefined
+ })
+ }}
+ model={items}
+ home={home}
+ />
+
+
+ >
+ );
+}
diff --git a/components/doc/breadcrumb/pt/wireframe.js b/components/doc/breadcrumb/pt/wireframe.js
new file mode 100644
index 0000000000..98803fb376
--- /dev/null
+++ b/components/doc/breadcrumb/pt/wireframe.js
@@ -0,0 +1,13 @@
+import React from 'react';
+import { DocSectionText } from '../../common/docsectiontext';
+
+export const Wireframe = (props) => {
+ return (
+ <>
+
+
+
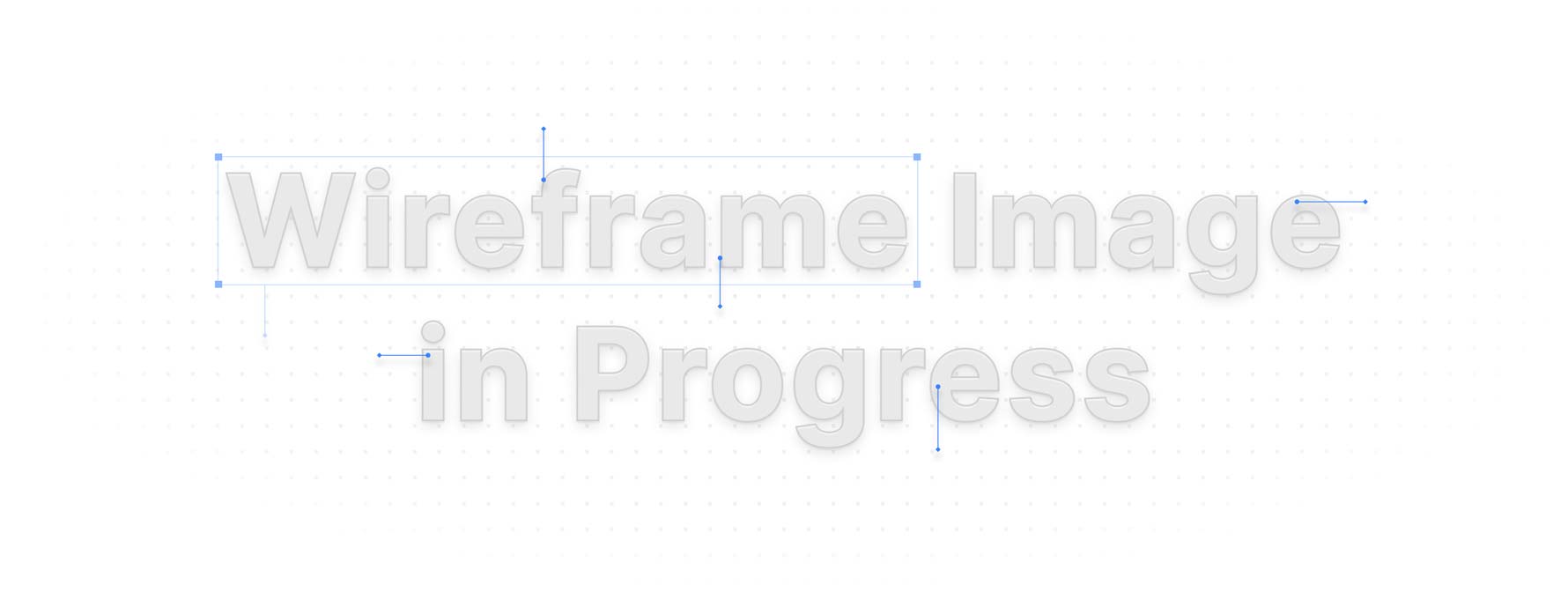
+
+ >
+ );
+};
diff --git a/components/doc/common/apidoc/index.json b/components/doc/common/apidoc/index.json
index 6165ba875f..35a7a4879f 100644
--- a/components/doc/common/apidoc/index.json
+++ b/components/doc/common/apidoc/index.json
@@ -3520,6 +3520,14 @@
"type": "ReactNode",
"default": "",
"description": "Used to get the child elements of the component."
+ },
+ {
+ "name": "pt",
+ "optional": true,
+ "readonly": false,
+ "type": "BreadCrumbPassThroughOptions",
+ "default": "",
+ "description": "Uses to pass attributes to DOM elements inside the component."
}
]
},
@@ -3528,6 +3536,88 @@
"values": []
}
}
+ },
+ "interfaces": {
+ "description": "Defines the custom interfaces used by the module.",
+ "values": {
+ "BreadCrumbPassThroughMethodOptions": {
+ "description": "Custom passthrough(pt) option method.",
+ "relatedProp": "",
+ "props": [
+ {
+ "name": "props",
+ "optional": false,
+ "readonly": false,
+ "type": "BreadCrumbProps"
+ }
+ ],
+ "callbacks": []
+ },
+ "BreadCrumbPassThroughOptions": {
+ "description": "Custom passthrough(pt) options.",
+ "relatedProp": "pt",
+ "props": [
+ {
+ "name": "root",
+ "optional": true,
+ "readonly": false,
+ "type": "BreadCrumbPassThroughType>",
+ "description": "Uses to pass attributes to the root's DOM element."
+ },
+ {
+ "name": "menu",
+ "optional": true,
+ "readonly": false,
+ "type": "BreadCrumbPassThroughType>",
+ "description": "Uses to pass attributes to the list's DOM element."
+ },
+ {
+ "name": "menuitem",
+ "optional": true,
+ "readonly": false,
+ "type": "BreadCrumbPassThroughType>",
+ "description": "Uses to pass attributes to the list item's DOM element."
+ },
+ {
+ "name": "action",
+ "optional": true,
+ "readonly": false,
+ "type": "BreadCrumbPassThroughType>",
+ "description": "Uses to pass attributes to the action's DOM element."
+ },
+ {
+ "name": "icon",
+ "optional": true,
+ "readonly": false,
+ "type": "BreadCrumbPassThroughType | SVGProps>",
+ "description": "Uses to pass attributes to the icon's DOM element."
+ },
+ {
+ "name": "label",
+ "optional": true,
+ "readonly": false,
+ "type": "BreadCrumbPassThroughType>",
+ "description": "Uses to pass attributes to the label's DOM element."
+ },
+ {
+ "name": "separatorIcon",
+ "optional": true,
+ "readonly": false,
+ "type": "BreadCrumbPassThroughType | SVGProps>",
+ "description": "Uses to pass attributes to the separator icon's DOM element."
+ }
+ ],
+ "callbacks": []
+ }
+ }
+ },
+ "types": {
+ "description": "Defines the custom types used by the module.",
+ "values": {
+ "BreadCrumbPassThroughType": {
+ "values": "PassThroughType"
+ }
+ }
}
},
"button": {
diff --git a/components/lib/breadcrumb/BreadCrumb.js b/components/lib/breadcrumb/BreadCrumb.js
index c360a0f1f5..f37a960555 100644
--- a/components/lib/breadcrumb/BreadCrumb.js
+++ b/components/lib/breadcrumb/BreadCrumb.js
@@ -1,12 +1,14 @@
import * as React from 'react';
-import { classNames, IconUtils, ObjectUtils } from '../utils/Utils';
+import { classNames, IconUtils, mergeProps, ObjectUtils } from '../utils/Utils';
import { BreadCrumbBase } from './BreadCrumbBase';
import { ChevronRightIcon } from '../icons/chevronright';
export const BreadCrumb = React.memo(
React.forwardRef((inProps, ref) => {
const props = BreadCrumbBase.getProps(inProps);
-
+ const { ptm } = BreadCrumbBase.setMetaData({
+ props
+ });
const elementRef = React.useRef(null);
const itemClick = (event, item) => {
@@ -38,14 +40,26 @@ export const BreadCrumb = React.memo(
const { icon: _icon, target, url, disabled, style, className: _className, template } = home;
const className = classNames('p-breadcrumb-home', { 'p-disabled': disabled }, _className);
- const icon = IconUtils.getJSXIcon(_icon, { className: 'p-menuitem-icon' }, { props });
-
- let content = (
- itemClick(event, home)}>
- {icon}
-
+ const iconProps = mergeProps(
+ {
+ className: 'p-menuitem-icon'
+ },
+ ptm('icon')
+ );
+ const icon = IconUtils.getJSXIcon(_icon, { ...iconProps }, { props });
+ const actionProps = mergeProps(
+ {
+ href: url || '#',
+ className: 'p-menuitem-link',
+ 'aria-disabled': disabled,
+ target,
+ onClick: (event) => itemClick(event, home)
+ },
+ ptm('action')
);
+ let content = {icon};
+
if (template) {
const defaultContentOptions = {
onClick: (event) => itemClick(event, home),
@@ -58,11 +72,15 @@ export const BreadCrumb = React.memo(
content = ObjectUtils.getJSXElement(template, home, defaultContentOptions);
}
- return (
-
- {content}
-
+ const menuitemProps = mergeProps(
+ {
+ className,
+ style
+ },
+ ptm('menuitem')
);
+
+ return {content};
}
return null;
@@ -70,8 +88,14 @@ export const BreadCrumb = React.memo(
const createSeparator = () => {
const iconClassName = 'p-breadcrumb-chevron';
- const icon = props.separatorIcon || ;
- const separatorIcon = IconUtils.getJSXIcon(icon, { className: iconClassName }, { props });
+ const separatorIconProps = mergeProps(
+ {
+ className: iconClassName
+ },
+ ptm('separatorIcon')
+ );
+ const icon = props.separatorIcon || ;
+ const separatorIcon = IconUtils.getJSXIcon(icon, { ...separatorIconProps }, { props });
return separatorIcon;
};
@@ -82,12 +106,24 @@ export const BreadCrumb = React.memo(
}
const className = classNames(item.className, { 'p-disabled': item.disabled });
- const label = item.label && {item.label};
- let content = (
- itemClick(event, item)} aria-disabled={item.disabled}>
- {label}
-
+ const labelProps = mergeProps(
+ {
+ className: 'p-menuitem-text'
+ },
+ ptm('label')
+ );
+ const label = item.label && {item.label};
+ const actionProps = mergeProps(
+ {
+ href: item.url || '#',
+ className: 'p-menuitem-link',
+ target: item.target,
+ onClick: (event) => itemClick(event, item),
+ 'aria-disabled': item.disabled
+ },
+ ptm('action')
);
+ let content = {label};
if (item.template) {
const defaultContentOptions = {
@@ -101,11 +137,15 @@ export const BreadCrumb = React.memo(
content = ObjectUtils.getJSXElement(item.template, item, defaultContentOptions);
}
- return (
-
- {content}
-
+ const menuitemProps = mergeProps(
+ {
+ className,
+ style: item.style
+ },
+ ptm('menuitem')
);
+
+ return {content};
};
const createMenuitems = () => {
@@ -138,15 +178,26 @@ export const BreadCrumb = React.memo(
getElement: () => elementRef.current
}));
- const otherProps = BreadCrumbBase.getOtherProps(props);
const className = classNames('p-breadcrumb p-component', props.className);
const home = createHome();
const items = createMenuitems();
const separator = createSeparator();
+ const menuProps = mergeProps(ptm('menu'));
+ const rootProps = mergeProps(
+ {
+ id: props.id,
+ ref: elementRef,
+ className,
+ style: props.style,
+ 'aria-label': 'Breadcrumb'
+ },
+ BreadCrumbBase.getOtherProps(props),
+ ptm('root')
+ );
return (
-